Compilation of a C program is a four stage process- Preprocessing (creates file.i ) Compilation (creates file.s ) Assembly (creates file.o ) Linking (creates executable file ) Note- when we will generate all intermediate files for a cpp program preprocessing generates file.ii while other are same as C program intermediate files. We will discuss details of these steps later.First of all make a .c or .cpp file- Open your terminal and type this command- gedit hello.c and write the following code in this file. After saving this type this command in your terminal- gcc -save-temps hello hello.c (for .c file) g++ -save-temps hello hello.cpp (for .cpp file) Now open your folder where you have created the progra...
Posts
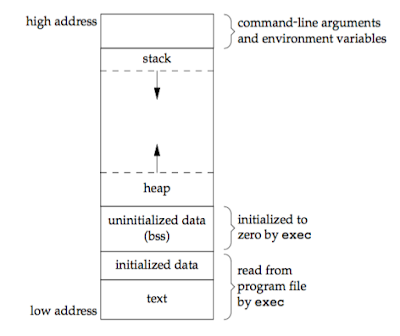
When we run C program it loaded into the ram in a very organized fashion.There are various segments to store code data and code instructions. The memory layout of a C program consist following sections- Text or code segment Initialized Data segment Uninitialized Data segment or .bss segment Heap segment Stack segment Command line argumets Text or Code segment - It is the segment in memory which contains executable instructions or compiled program(./a.out or .exe file). Usually, the text segment is sharable so that only a single copy needs to be in memory for frequently executed programs, such as text editors, the C compiler, the shells, and so on. Also, the text segment is often read-only, to prevent a program from accidentally modifying its instructions. Initialized data segment- It is basically a data segment which contains all global , static , constant , external (variable with extern keyword) variables that are initialized in the program. This is not just a read-...
Definition- structured programming does not means programming with structure.It is a programing done by using type of code structures given below- 1-Sequence of sequentially executed statements 2-Conditioanal execution statements (i.e. If ,Else) 3-Looping or iterations (i.e.For,do..while,while) 4-Structured subroutine calls (Functions) Advantage of structured programming- 1-Complexity can be reduced by dividing the problem in substructures. 2-Structure will give us clear control to the problem 3-Substructures or modules can be used repeatedly 4-Easy to update and add modules Disadvantage of structured programming- The main disadvantage of this style of programming is that all decisions are to be made from the start of the project and we know that specification changes by the time so whenever we need to change specification we have a great risk of rewriting a large part of project.

Definition- A header linked list is a type of linked list which always contains a special node called the header node at the very beginning of the linked list.It is an extra node kept at the front of a list. Such a node does not represent an item in the linked list.The information part of this node can be used to store any global information about the whole linked list. Source- pages.cs.wisc.edu Advantages of having a header node- In header node, you can maintain count variable which gives number of nodes in the list. You can update header node count member whenever you add /delete any node. It will help in getting list count without traversing in O(1) time. In addition to address of first node you can also store the address of last node. So that if you want to insert node at the rear end you don't have to iterate from the beginning of the list. Also in tcase of DLL(Doubly Linked List) if you want to traverse from rear end it helps. We can also use it to store the poi...

DDA algorithm is the basic algorithm for line drawing.In this method, we start from the starting point and then on each step a fixed increment is added to the current point to get the next point on the line.We repeat these steps till the end of the line. Let us suppose (x1,y1) and (x2,y2) are the end points of the line and the equation of the line is y=mx+c. slope of the line=(y2-y1)/(x2-x1) suppose dx=(x2-x1) and dy=(y2-y1) dx=dy/m and dy=m*dx dx and dy will be the values for incrementing x and y values of current point.so- X i+1 =X i + dy/m Y i+1 =Y i + m*dx DDA Algorithm- Read the points (x...

In Mid-point Ellipse drawing algorithm we use 4 way symmetry of the ellipse to generate it.We perform calculations for one part and the other three parts will be drawn by using 4-way symmetry. For given parameter Rx and Ry (radii of ellipse) and (Xc,Yc)-center of ellipse, we determine the point (x,y) for an ellipse in standard position centered on the origin and then we shift the points so the ellipse is centered at (Xc,Yc). We can see the image given below, how we choose next point. Source- www.slideshare.net Algorithm- Read the two radii Rx and Ry Initialize starting point x=0,y=Ry Calculate initial decision variable for region 1 d1=Ry*Ry-Rx*Rx*Ry-(Rx*Rx)/4 Region 1 do{ plot(x,y) and other 3 symmetric points if(d1<0) ...

Transformation of any object in a 2D or 3D plane means the change in the looks of the object by applying certain rules. Scaling- It is one of the transformation technique. Scaling changes the size of an object.This operation is carried out in any polygon by multiplying the scaling factor (Sx,Sy) with each vertex coordinate value (x,y) of the polygon.This results in transform coordinates (x',y') and then we plot the transformed coordinates. Here is the program to scale a triangle so Let's suppose (x1,y1) (x2,y2) (x3,y3) are the coordinates of a triangle and the scaling factor is (Sx,Sy) then transform coordinates will be- (x1',y1')=(Sx*x1,Sy*y1) (x2',y2')=(Sx*x2,Sy*y2) (x3',y3')=(Sx*x3,Sy*y3) One thing you need to keep in mind that th...

Flood Fill Algorithm- Flood fill algorithm is used to color the polygon.This comes under seed fill algorithm.To color a polygon we use either 4-connected method or 8-connected method. 4-connected method- In this method, we choose a seed pixel and color it with new color after this we color the neighbourhood pixels and so on. 8-connected method- In this method, we choose a seed pixel and color it with new color after this we color all eight neighbourhood pixels and so on. we can see this by this picture- Source-https://www.cs.auckland.ac.nz/courses/compsci773s1c/lectures/ImageProcessing-html/neighbourhoods.gif Here is the c++ program for flood fill algorithm- #include<bits/stdc++.h> #include<graphics.h> using namespace std; //function to implement floodfill algorithm void floodfill(int x,int y,int old,int newcol) { //getpixel() gives the color of pixel (x,y) int curr=getpixel(x,y); //if the color of current pixel is same as the old color then color this to new colo...